Question: 1
Given the code fragment:
class CallerThread implements Callable {
String str;
public CallerThread(String s) {this.str=s;}
public String call() throws Exception {
return str.concat(''Call'');
}
}
and
public static void main (String[] args) throws InterruptedException, ExecutionException
{
ExecutorService es = Executors.newFixedThreadPool(4); //line n1
Future f1 = es.submit (newCallerThread(''Call''));
String str = f1.get().toString();
System.out.println(str);
}
Which statement is true?
Question: 2
Given the code fragment:
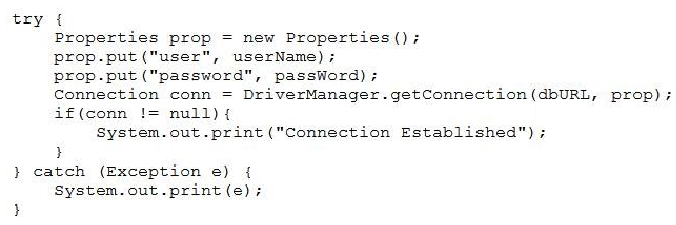
and the information:
The required database driver is configured in the classpath.
The appropriate database is accessible with the dbURL, username, and passWord exists.
What is the result?
Question: 3
Given the definition of the Vehicle class:
Class Vehhicle {
int distance; //line n1
Vehicle (int x) {
this distance = x;
}
public void increSpeed(int time) { //line n2
int timeTravel = time; //line n3
class Car {
int value = 0;
public void speed () {
value = distance /timeTravel;
System.out.println (“Velocity with new speedâ€+value+â€kmphâ€);
}
}
new Car().speed();
}
}
and this code fragment:
Vehicle v = new Vehicle (100);
v.increSpeed(60);
What is the result?
Question: 4
Given:
IntStream stream = IntStream.of (1,2,3);
IntFunction inFu= x -> y -> x*y; //line n1
IntStream newStream = stream.map(inFu.apply(10)); //line n2
newStream.forEach(System.output::print);
Which modification enables the code fragment to compile?
Question: 5
Given the definition of the Country class:
public class country {
public enum Continent {ASIA, EUROPE}
String name;
Continent region;
public Country (String na, Continent reg) {
name = na, region = reg;
}
public String getName () {return name;}
public Continent getRegion () {return region;}
}
and the code fragment:
List couList = Arrays.asList (
new Country (''Japan'', Country.Continent.ASIA),
new Country (''Italy'', Country.Continent.EUROPE),
new Country (''Germany'', Country.Continent.EUROPE));
Map> regionNames = couList.stream ()
.collect(Collectors.groupingBy (Country ::getRegion,
Collectors.mapping(Country::getName, Collectors.toList()))));
System.out.println(regionNames);