Question: 1
Given the structure of the Student table:
Student (id INTEGER, name VARCHAR)
Given the records from the STUDENT table:
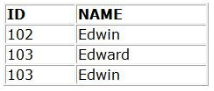
Given the code fragment:

Assume that:
The required database driver is configured in the classpath.
The appropriate database is accessible with the dbURL, userName, and passWord exists.
What is the result?
Question: 2
Given the code fragment:
UnaryOperator<Integer> uo1 = s -> s*2;line n1
List<Double> loanValues = Arrays.asList(1000.0, 2000.0);
loanValues.stream()
.filter(lv -> lv >= 1500)
.map(lv -> uo1.apply(lv))
.forEach(s -> System.out.print(s + '' ''));
What is the result?
Question: 3
Given the definition of the Emp class:
public class Emp
private String eName;
private Integer eAge;
Emp(String eN, Integer eA) {
this.eName = eN;
this.eAge = eA;
}
public Integer getEAge () {return eAge;}
public String getEName () {return eName;}
}
and code fragment:
List<Emp>li = Arrays.asList(new Emp(''Sam'', 20), New Emp(''John'', 60), New Emp(''Jim'', 51));
Predicate<Emp> agVal = s -> s.getEAge() > 50;//line n1
li = li.stream().filter(agVal).collect(Collectors.toList());
Stream<String> names = li.stream()map.(Emp::getEName);//line n2
names.forEach(n -> System.out.print(n + '' ''));
What is the result?
Question: 4
Given the code fragment:
List<String> codes = Arrays.asList (''DOC'', ''MPEG'', ''JPEG'');
codes.forEach (c -> System.out.print(c + '' ''));
String fmt = codes.stream()
.filter (s-> s.contains (''PEG''))
.reduce((s, t) -> s + t).get();
System.out.println(''\n'' + fmt);
What is the result?
Question: 5
Given the code fragment:
public class Foo {
public static void main (String [ ] args) {
Map<Integer, String> unsortMap = new HashMap< > ( );
unsortMap.put (10, ''z'');
unsortMap.put (5, ''b'');
unsortMap.put (1, ''d'');
unsortMap.put (7, ''e'');
unsortMap.put (50, ''j'');
Map<Integer, String> treeMap = new TreeMap <Integer, String> (new
Comparator<Integer> ( ) {
@Override public int compare (Integer o1, Integer o2) {return o2.compareTo
(o1); } } );
treeMap.putAll (unsortMap);
for (Map.Entry<Integer, String> entry : treeMap.entrySet () ) {
System.out.print (entry.getValue () + '' '');
}
}
}
What is the result?